Function Decoration: Insights and Practical Applications
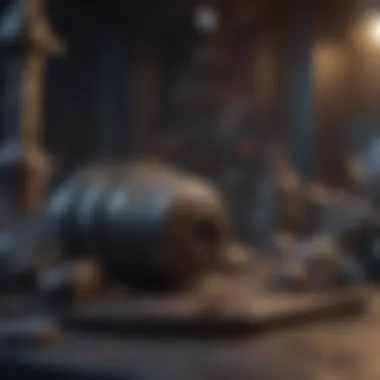
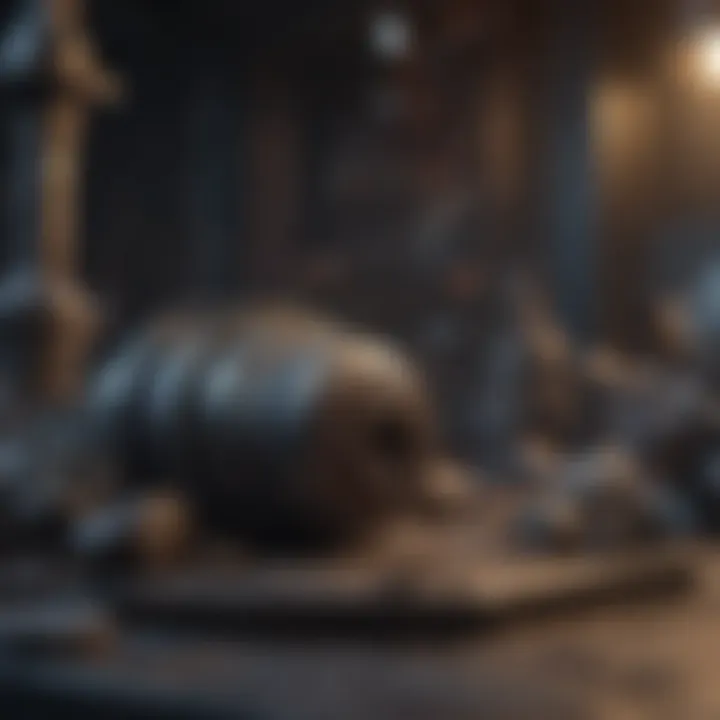
Intro
Function decoration is crucial in modern programming. It allows developers to enhance existing functions without modifying their core structure. This technique becomes vital for maintainability and scalability. Many programming languages support this concept, allowing programmers to use decorators effectively.
This article aims to unpack the principles, applications, and implications surrounding function decoration. It serves not only to inform but to highlight how this technique can be utilized for improved software design, especially for students, researchers, educators, and professionals.
Research Highlights
Key Findings
- Flexibility and Reusability: Function decoration provides a flexible approach to modify behavior. Developers can easily apply the same decoration to multiple functions, promoting code reusability.
- Improved Maintainability: By separating concerns through decorators, codebases become easier to manage. This separation allows for clearer logic within functions.
- Real-World Applications: Function decoration has been successfully applied in various frameworks like Flask and Django. These applications showcase how decorators enrich the functionality of web applications.
"Function decorators elevate code quality and keep software structures clean and understandable."
Implications and Applications
- Enhancing Security: Through decorators, developers can enforce security checks. For example, decorators can validate user access before executing certain functions.
- Logging and Monitoring: Using decorators for logging purposes can help keep track of function calls and their performance metrics, which is invaluable for debugging.
- Framework Integration: In web development, decorators streamline routing and middleware functions, integrating seamlessly with frameworks. This practical application shows their importance in developing robust web services.
Methodology Overview
Research Design
This exploration of function decoration is based on a qualitative approach. By analyzing coding practices and existing literature, it aims to provide a comprehensive understanding.
Experimental Procedures
Interviews and surveys among developers were conducted. This provided insights into common practices and challenges faced when implementing decorators in their projects. The feedback helped highlight areas where function decoration excels and where it may present difficulties.
Using tools like GitHub, researchers collected real-world examples where decorators were successfully implemented and cases where developers encountered pitfalls.
By synthesizing this information, the article will discuss best practices to enhance the use of function decoration.
Understanding function decoration fundamentally shifts how developers approach coding tasks. The benefits are tangible, making it an essential topic worthy of deep exploration.
Understanding Function Decoration
Understanding function decoration is crucial in grasping the broader landscape of modern programming practices. This concept serves as a functional technique that allows developers to enhance existing functions with additional capabilities, without modifying their core logic. By delving into function decoration, one can appreciate its role in increasing code reusability, flexibility, and overall maintainability. In an era where software complexity is ever-growing, such techniques become invaluable, ensuring that developers can implement features seamlessly while adhering to best coding practices.
Definition and Overview
Function decoration is a method used primarily in programming languages like Python, where a decorator is a function that takes another function and extends its behavior without modifying its actual code. This is achieved by wrapping the original function inside a new function. The decorator can add pre-processing and post-processing actions before and after the original function executes. This layering of functionality is a powerful approach to structuring code.
In simple terms, decorators provide a syntactical shortcut for modifying the behavior of a function or class. For example, through the use of the syntax in Python, a function can be easily decorated. This enhances both clarity and intent, allowing a developer to quickly grasp the additional functionalities assigned to functions.
Historical Context
The idea of decorators finds its roots in the broader discussion on higher-order functions. Higher-order functions are those that can take other functions as arguments or return them as results. This concept is not new. It has been present in functional programming languages for decades. However, its adoption in mainstream languages like Python and JavaScript has facilitated wider exploration.
Python first introduced decorators in the early 2000s, providing programmers with a novel way to tackle common problems in a clean and Pythonic manner. As programming paradigms continue to evolve, decorators have emerged as a go-to solution for enhancing functionalities in a modular way. Their utility in handling cross-cutting concerns, such as logging and authentication, underlines their significance within the development community.
Understanding these elements not only emphasizes the practical benefits of function decoration but also contextualizes its evolution within programming languages. The integrative approach of function decoration represents a significant advance in how developers can manage complexity while fostering cleaner, more efficient code.
Core Principles of Function Decoration
Understanding the core principles of function decoration is crucial for anyone looking to enhance their coding skills. Function decoration stands as a powerful tool in programming, particularly in languages such as Python. The principles provide a framework that ensures decorators can be applied effectively, promoting clearer code and improved functionality.
The Concept of Higher-Order Functions
Higher-order functions are fundamental to the concept of function decorators. In programming, a higher-order function is any function that takes one or more functions as arguments or returns another function as its output. This property allows decorators to wrap another function, adding new behavior or altering its output.
In practical terms, a decorator can be seen as a type of higher-order function. For example, consider a simple scenario where you want to log the execution time of a function. By creating a higher-order function that wraps your original function, you can measure its runtime without modifying the function itself. Here’s a brief illustration:
In this code, is a higher-order function that wraps . This approach keeps your code modular and clean, making debugging and maintenance more straightforward.
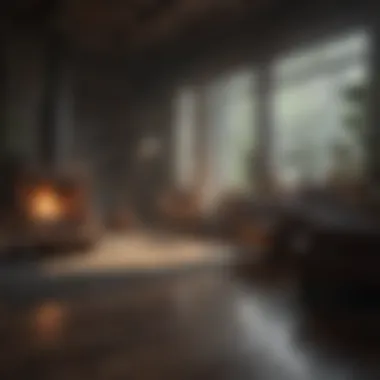
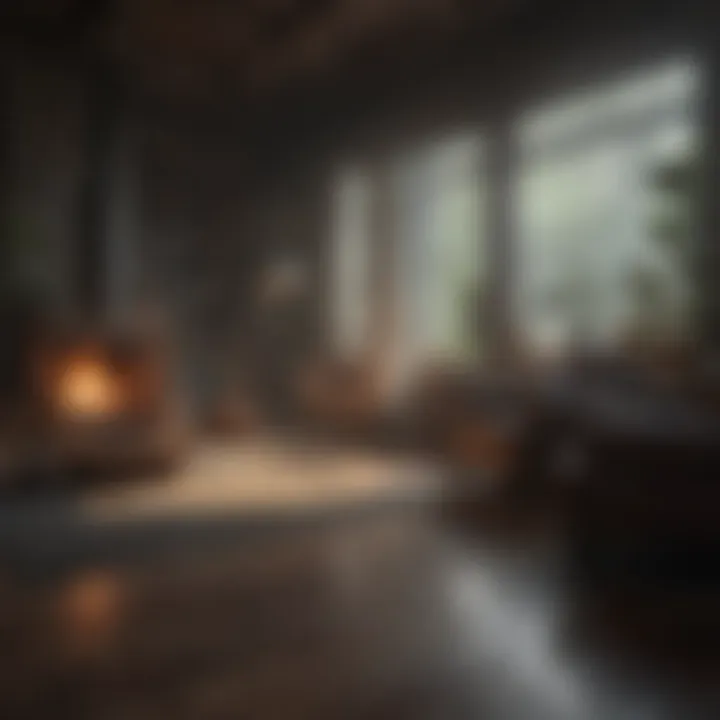
Closure Mechanics
Closures are a key aspect of function decorators. A closure occurs when a function retains access to its lexical scope, even when that function is executed outside its original scope. This concept is instrumental when designing decorators, as it allows decorators to maintain state or context.
When a decorator is applied, it typically uses a nested function to capture and utilize information from the outer function. Consider this:
In this example, the uses a closure to keep track of how many times has been called. Each time it's invoked, the function updates and retains the count, showcasing how closures enhance the capabilities of decorators.
In summary, recognizing the roles of higher-order functions and closures forms the bedrock of function decoration. These principles empower programmers to create flexible and sophisticated code structures. Embracing them can lead to well-organized, efficient, and highly maintainable code.
Applications of Function Decoration
The application of function decoration is a pivotal aspect of modern programming practices. It allows developers to alter or enhance the behavior of functions effortlessly. This is especially relevant in environments that demand modularity and clarity in code. The flexibility and reusability of decorated functions can lead to more concise, readable, and maintainable code. Engaging with these applications not only optimizes performance but also aligns with best programming practices.
Enhancing Functionality
Function decoration plays a crucial role in enhancing functionality. By using decorators, developers can wrap additional behaviors around existing functions without modifying their core logic. For example, one can add timing features to track how long a function takes to execute or apply memoization to cache results from resource-heavy computations.
The ability to apply such enhancements directly through decorators keeps the code clean. Instead of cluttering function definitions with various functionalities, we can keep them separate. This separation of concerns aids in clarity, making it easier to understand and maintain the codebase.
In this example, the timer_decorator wraps the compute_square function. Every time compute_square is called, the timer_decorator will record how long the execution takes. This enhances functionality without altering the logic of compute_square.
Logging and Monitoring
Logging and monitoring are vital in software applications. Function decorators can streamline these tasks. By implementing a logging decorator, developers can automatically log function calls, their parameters, and returned values. This helps in tracking the flow of the application and diagnosing issues effectively.
For instance, a simple logging decorator can be implemented as follows:
Here, the logging_decorator captures the function call's input and output, offering insights during development and production environments. Monitoring the function's execution allows developers to identify unexpected behaviors or errors more readily.
Authorization Checks
In security-critical applications, function decorators serve as an ideal solution for authorization checks. By utilizing decorators to enforce permission controls, developers can ensure that only authorized users can trigger specific functions. This approach centralizes the authentication logic, promoting consistency and reducing code duplication.
For example:
In this snippet, the require_authentication decorator checks if a user is authenticated. If not, it raises an exception, effectively preventing unauthorized access to sensitive operations. This design pattern ensures that security checks are consistently applied across functions without embedding logic within them.
Function decoration provides powerful solutions for enhancing functionality, logging, and access control. By employing decorators, developers can maintain cleaner code, improve security, and foster better monitoring practices.
The Syntax of Function Decoration
Understanding the syntax of function decoration is fundamental in grasping how decorators function within programming. It provides the backbone for creating efficient, dynamic, and reusable code snippets. Utilizing decorators correctly can elevate code readability, speed up development time, and provide powerful functionality without cluttering the primary function body's logic.
When discussing syntax, it is crucial to examine the various components involved in constructing decorators. This includes understanding how decorators are defined, how they wrap functions, and how they are applied to achieve the desired effects. The clarity of the syntax directly influences how developers conceptualize and implement these concepts in real-world applications.
Basic Syntax Overview
The basic syntax of a decorator in programming is straightforward yet powerful. It typically follows a specific structure, composed of a function that returns another function. Here is a simple overview of the basic syntax:
- Define a decorator function: This is the function that will take another function as its argument. It is usually nested within another function, creating the closure required for decoration.
- Use the decorator: The decorator is then applied to a function using the syntax before the function definition.
Here is a coded example to illustrate this:
In the example above, the function takes as an argument, enhancing its functionality with additional print statements. This separation maintains the intention of while adding another layer of behavior.
Instantiation of Decorators
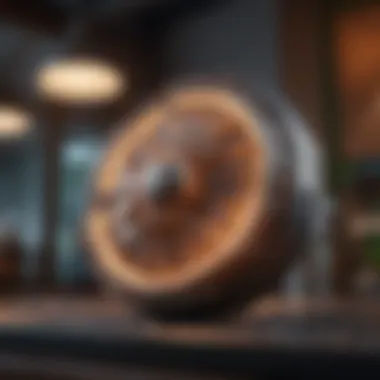
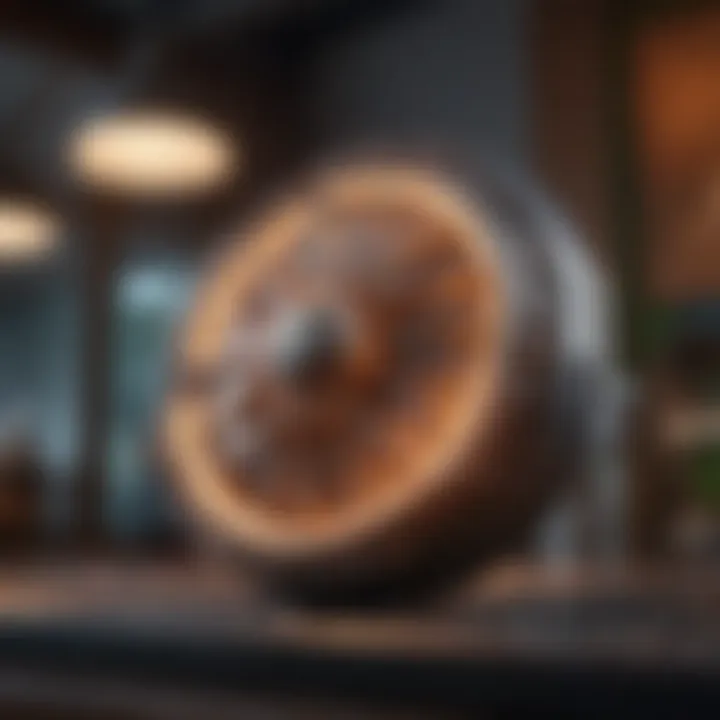
Once a decorator is defined, instantiation is a critical next step. This process involves applying the decorator to a function, effectively replacing it with the wrapped version. The syntax for instantiation is simple, yet understanding its implications is vital.
When decorating a function, the original function is replaced by the function returned from the decorator.
- Direct application: As shown in the previous example, the decorator can be directly applied above the function definition.
- Manual application: Alternatively, a function can be wrapped after its definition by calling the decorator explicitly.
Example:
This alternative method of instantiation allows for more dynamic usage of decorators, enabling conditional application and greater flexibility in programming.
The use of decorators leads to cleaner code and enhances maintainability and readability. It's essential to use them wisely and appropriately.
By mastering these elements of syntax, developers can unlock the full potential of function decoration, making their code more modular and easier to manage as requirements evolve.
Examining Decorator Patterns
Examining decorator patterns is crucial in understanding the versatility of function decoration. These patterns illustrate how decorators can enhance functionality while keeping the core logic intact. By analyzing various patterns, developers can choose appropriate strategies when implementing decorators. This section focuses on single decorators and chaining multiple decorators, providing insight on their uses and advantages.
Single Decorators
Single decorators serve as the foundational building blocks of function decoration. These decorators wrap a specific function to add functionality without modifying its code. They can handle various tasks such as logging, validation, and measurement of execution time.
Using a single decorator is straightforward. It modifies a function's behavior in a clean and efficient manner. For instance, a decorator could log the entry and exit points of a function. The implementation might look like this:
In this code, the decorator adds logging capabilities to a function, illustrating the essence of a single decorator—maintaining simplicity while enhancing functionality. When using a single decorator, focus on clarity and purpose. This helps maintain code readability and ensures other developers can easily understand the codebase.
Chaining Multiple Decorators
Chaining multiple decorators introduces additional complexity but also more power. This approach allows several decorators to be applied in a stacked manner, enhancing a function with different capabilities. Each decorator processes the output of the previous one, creating a cumulative effect.
When chaining decorators, it is essential to consider the order in which they are applied. The stacking sequence affects behavior. For instance, if an authorization check decorator is applied after a logging decorator, the logging may document unauthorized access attempts.
A simple example of chaining multiple decorators is shown below:
In this example, first checks for authorization and then logs the execution. The clarity of each decorator’s purpose is crucial when chaining to maintain a clean and understandable code structure.
Key Considerations
- Prioritize readability when chaining decorators.
- Ensure each decorator has a clearly defined purpose.
- Regularly review the interaction of combined decorators to prevent unintended side effects.
Chaining multiple decorators can significantly enrich a function’s behavior. Developers must be mindful of how these decorators work together to avoid making the codebase overly complex. Keeping these practices at the forefront supports maintainability and extensibility in future developments.
Best Practices in Implementation
The implementation of function decoration is not just about mastering syntax; it involves carefully considering the aspects that influence maintainability and clarity. Best practices provide guidelines that enhance the effectiveness of decorators, ensuring that they serve their intended purpose without causing unintended complexities. By following these practices, developers can create cleaner, more efficient code, ultimately leading to a more robust software.
Naming Conventions
Naming is crucial in programming, and this holds true for decorators as well. Clear and descriptive naming conventions make it easier for developers to understand a decorator’s function at a glance. Here are some points to consider when naming decorators:
- Be Descriptive: Names should convey what the decorator does. For example, a decorator named makes it easy to grasp its purpose compared to a generic name like .
- Use Consistent Patterns: Consistency aids comprehension. If you use a particular verb-noun pattern for naming, stick with it across your codebase.
- Avoid Abbreviations: While abbreviations might save characters, they can also obscure meaning. It is better to err on the side of clarity.
- Indicate Side Effects: If a decorator has side effects, this should be reflected in its name. For instance, indicates that the function performs both logging and user authentication.
By adopting these naming conventions, you promote clarity in your code and help team members quickly grasp the functionality of various decorators.
Keeping Decorators Simple
Simplicity is a cornerstone of effective function decoration. Decorators should add specific functionality without overwhelming the core logic of the function they modify. Below are essential considerations for maintaining simplicity:
- Single Responsibility Principle: Decorators should aim to accomplish one task. A decorator that does too much can become difficult to manage and understand. For example, instead of mixing logging, authentication, and error handling, create separate decorators for each.
- Limit Nested Decorators: While chaining decorators can be powerful, excessive nesting can lead to confusion. Always assess whether chaining is truly necessary.
- Clear Logic Flow: Ensure that the logic within a decorator is straightforward. Complicated logic may obfuscate the function's purpose, making it hard to debug.
- Commenting: Adding comments within the decorator can guide other developers through its functionality. This is especially useful for complex decorators.
By keeping decorators simple, developers can avoid common pitfalls, such as those related to complexity and maintainability.
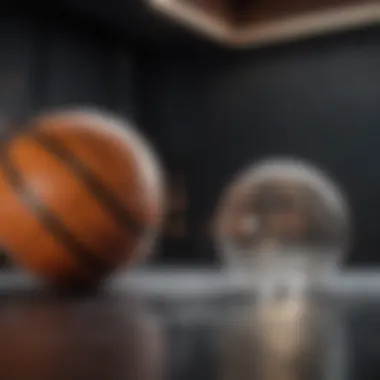
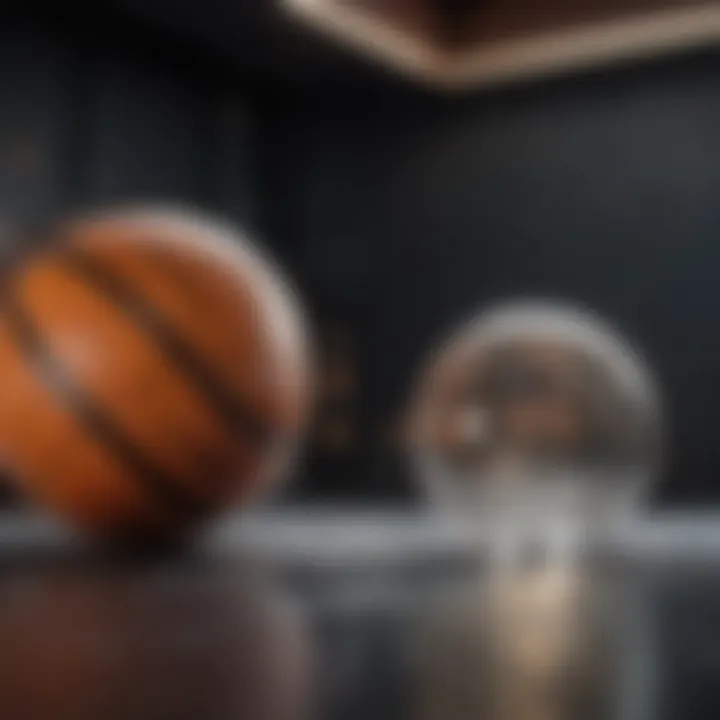
Simplicity in design enhances the reliability of code, making it easier to test and support over time.
Using best practices in implementation fosters greater efficiency and understanding of function decoration, creating a culture of clarity in collaborative environments. Those who understand the principles of naming and simplicity engage more effectively in code review and maintenance.
Potential Pitfalls in Function Decoration
Understanding the potential pitfalls in function decoration is essential for developers and programmers. While function decoration offers various benefits such as code reusability and enhanced functionality, it also introduces challenges that can affect the maintainability and overall structure of the code. In this section, we discuss critical aspects that one must consider when implementing decorators in a programming environment.
Complexity and Maintainability Issues
Function decorators can significantly increase the complexity of a codebase. When multiple decorators are applied to a single function, tracking the flow of execution becomes challenging. This situation can obscure the logical structure of the code and lead to confusion. As decorators alter function behavior, understanding what each decorator does, especially in chained scenarios, is crucial for developers.
For example, when a function has several decorators, the execution order can affect the output. If a decorator performs modifications before another one is applied, the final result can be different than expected.
- Clarity: Maintaining clarity is essential. If decorators are complex or nested, new developers may struggle to comprehend the function's purpose and behavior. Simplicity is key.
- Documentation: Adequate documentation becomes critical in this scenario. Each decorator needs clear comments detailing its purpose and implementation.
- Refactoring: Over time, as applications grow, refactoring becomes necessary. Complex decorators may hinder this process, making it difficult to isolate issues or changes.
Debugging Challenges
Debugging decorated functions presents unique challenges. Since decorators encapsulate the function block, any errors that arise may be obscured, as they can be attributed to the decorator rather than the core function itself. This behavior can lead to difficulties in pinpointing the source of bugs.
- Error Tracking: When a function decorated with multiple layers fails, identifying the exact point of failure can be complicated. This situation requires deeper insight into each decorator's function.
- Stack Traces: Stack trace information may not always show the decorator's name correctly, making it harder to diagnose issues. A lack of specificity in error messages creates unnecessary delays in fixing the problem.
- Testing: Comprehensive testing needs to account for all decorators involved, which can extend testing time and complicate the testing framework.
Overall, while function decoration provides substantial advantages in software development, recognizing and managing its pitfalls is imperative. Developers should prioritize simplicity, clarity, and effective error management to facilitate a smooth coding experience.
Real-World Case Studies
In the realm of programming, real-world case studies serve as vital illustrations of how theoretical concepts are applied within practical settings. Understanding function decoration through these case studies provides insights into its benefits and challenges in diverse domains.
Real-world applications not only validate the principles of function decoration but also reveal its implications in actual development scenarios. Case studies bring clarity to abstract concepts, demonstrating how function decorators enhance functionality, streamline processes, or address specific problems. Furthermore, they emphasize the versatility and adaptability of these techniques across varying fields—setting a solid foundation for both current practitioners and future developers.
Function Decoration in Web Development
Web development stands as one of the prominent areas where function decoration plays a critical role. Frameworks like Flask and Django leverage decorators to manage routes, authentication, and middleware. These decorators provide an efficient way to wrap functionality around web application features.
For example, in Flask, function decorators are utilized to map URLs to specific functions. The decorator links a function to the Home endpoint of a web application. This not only reduces redundancy but also enhances readability.
Another significant application is in managing user permissions. Decorators can enforce security checks, ensuring that users only access the resources they are authorized to use. This simplifies code structure and reduces the likelihood of introducing errors.
Key Benefits
- Modularity: Decorators promote a modular approach, allowing developers to implement features independently.
- Easier Maintenance: Functions can be enhanced or modified without altering the core logic.
- Readability: Decorators clarify intent and usage within the code, making it easier to follow.
Function Decoration in Data Science
In the field of data science, decorators provide useful enhancements that allow for cleaner and more efficient code. For instance, preprocessing functions in machine learning can be decorated to automate tasks like data validation or transformation. Functions such as can be used to log the execution time of algorithms, providing valuable insights into performance.
Using decorators for data transformations also improves code maintainability. A decorator can be created to ensure that data is properly formatted before processing, reducing the chances of encountering errors down the line.
Specific Instances
- Performance Tracking: An example of function decoration is the implementation of performance tracking in a data pipeline, which helps monitor and optimize execution times.
- Data Cleaning: A decorator can be designated for data cleaning procedures, ensuring that datasets are always validated before use.
Real-world applications consistently demonstrate how function decoration offers practical advantages. They enhance clarity, simplify code, and foster more efficient designs in both web development and data science.
Epilogue
In concluding this exploration of function decoration, it is vital to highlight several key aspects. Function decoration simplifies code reuse and enhances modularity in programming. Through the encapsulation of functionality, decorators provide a means to augment existing functions without modifying their core behavior. This approach streamlines processes such as logging, authentication, and access control, making the workflow more efficient and easier to manage.
Ultimately, the benefits of function decoration lie in its ability to promote cleaner, more readable code. With appropriate use, developers can avoid redundancy and improve maintainability, translating into significant long-term advantages.
Summarizing Key Points
- Concept of Function Decoration: Function decoration allows users to extend the behavior of functions in a simple way, producing robust code structures.
- Higher-Order Functions: Decorators fall under the category of higher-order functions, which accept functions as arguments or return them.
- Practical Applications: They find use in various programming scenarios, including web development and data science, providing essential enhancements.
- Syntax and Patterns: Understanding the syntax and patterns of decorators is crucial for proper implementation. This includes knowing how to instantiate and chain decorators effectively.
- Best Practices: Adhering to best practices, such as using appropriate naming conventions and maintaining simplicity, reduces complexity and promotes maintainability in code.
- Potential Pitfalls: Awareness of challenges, such as debugging difficulties and possible convolutions in code structure, is essential for effective use.
Future Implications of Function Decoration
The future of function decoration appears promising as languages evolve to embrace more functional paradigms. As developers seek out cleaner and more maintainable code solutions, function decoration may become increasingly integral to software design. One potential trend includes the expansion of decorator functionality in popular programming frameworks, allowing for even greater adaptability.
Moreover, with the rise of artificial intelligence and machine learning, leveraging decorators may streamline data processing and model management. As decorators advance, understanding their implications on code organization and performance will be crucial. This anticipation suggests that a renewed focus on efficient architecture will emerge, making decorators an essential skillset for developers in the years to come.
"Function decorators enhance readability and structure. They are not just a coding gimmick; they represent a philosophy of clean, maintainable development."
In summary, the exploration of function decoration offers essential insights into coding practices. For students, researchers, educators, and industry professionals, mastering decorators not only improves immediate coding tasks but also prepares one for future advancements in programming methodologies.